Programming the .NET Framework 4.5
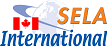

Description
This five-day instructor-led course provides students with the knowledge and skills to develop applications with the .NET Framework 4.5.
Developing applications for the .NET Framework 4.5 requires familiarity with fundamental mechanisms such as Garbage Collection, Serialization, Streams and Application Domains; integrating these applications into solutions written using other programming languages requires Interoperability; taking advantage of the latest hardware requires Multithreading and Asynchronous Programming. These skills are also required for taking advantage of application frameworks on top of the core CLR such as Windows Communication Foundation (WCF), Language Integrated Query (LINQ) and others.
The course is packed with practical code samples, demos and exercises to facilitate understanding the covered features from a .NET perspective, as well as from a more holistic system-oriented point of view.
Intended audience
This course is intended for developers with working knowledge of the C# 3.0 programming language.
▼Expand All
-
Module 1: Introduction
-
Overview of the .NET Framework
-
Overview of the .NET Type System
-
Visual Studio and .NET Framework Versions
-
.NET Backwards and Forwards Compatibility
-
Visual Studio Multi-Targeting Support
-
-
Module 2: Memory Management
-
Overview of Memory Management
-
Garbage Collection First Steps
-
GC Flavors
-
Generations
-
Interacting with the GC
-
Weak References
-
Finalization and the Dispose Pattern
-
Lab 1: Weak Timer
-
-
Module 3: Streams and File I/O
-
Streams as a Data Abstraction
-
File Streams
-
Stream Readers / Writers
-
The File and Directory Classes
-
Lab 2: Word Count
-
-
Module 4: Serialization
-
Motivation for Serialization
-
Marking a Type for Serialization
-
BinaryFormatter
-
Controlling Serialization
-
Custom Serialization
-
Overview of XML Serialization
-
Overview of DataContract Serialization
-
Lab 3: Serialization Framework
-
-
Module 5: Threading and Asynchronous Programming
-
Taxonomy of Multi-Threading
-
The Asynchronous Programming Model (APM)
-
Thread Pool
-
Manual Threading
-
Synchronization
-
Overview of Parallel Extensions for .NET
-
Lab 4: Picture Feed
-
Lab 5: Queuing Work
-
-
Module 6: Application Domains
-
Application Domains as Isolation Boundaries
-
Creating and Unloading AppDomains
-
Executing Code in an AppDomain
-
AppDomain Boundaries
-
Overview of .NET Remoting
-
Lab 6: Plug-in Framework
-
-
Module 7: Interoperability
-
Platform Invoke
-
COM Interoperability
-
C++/CLI
-
Lab 7: P/Invoke and Reverse P/Invoke
-
Lab 8: COM Interoperability (Optional)
-
Lab 9: C++/CLI Native to Managed
-
Lab 10: C++/CLI Managed to Native
-
-
Module 8: Advanced Topics
-
Improving Startup Performance with NGEN
-
Advanced Delegates and Events
-
Advanced Generics
-
Object Cloning as Serialization
-
Assembly Loading Problems and Contexts
-
Code Contracts
-
-
Module 9: Overviews
-
ADO.NET
-
System.Transactions
-
Windows Communication Foundation (WCF)
-
Windows Workflow Foundation (WF)
-
Language Integrated Query (LINQ)
-
Task Parallel Library (TPL)
-
New .NET 4.5 BCL Types
-
Related Courses
-
- Working knowledge of the C# 3.0 programming language,
- -or- Completed Course 50150: C# 5.0 Programming in the .NET Framework
- Develop applications that correctly interact with the .NET Garbage Collector;
- Use streams to read and write various data sources including files and memory buffers;
- Serialize and deserialize object data to different formats;
- Leverage hardware advances by using threads, thread pools, background workers and the Asynchronous Programming Model (APM);
- Isolate applications and plug-ins into separate Application Domains;
- Integrate .NET programs into mixed-language applications;
- Describe other application frameworks (such as WCF and LINQ) on top of the core CLR.
Contact Us


SEND
Related Courses