LINQ via C#
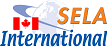

Description
This three-day instructor-led course provides students with the knowledge and skills to develop applications with the C# 3.0 language and the LINQ (Language Integrated Query) framework.
LINQ is Microsoft’s framework for bridging the gap between various data sources and the .NET object-oriented languages. LINQ abstracts away the data source, providing an intuitive, declarative and uniform query interface to object collections, SQL-based relational databases and XML documents. Its extensibility features facilitate integrating additional data sources through the use of custom query providers. LINQ C# integration relies heavily on a set of new features of the C# 3.0 language.
The course is packed with practical code samples, demos and exercises to facilitate understanding the motivation of LINQ, the design patterns for its application, and its potential pitfalls.
Intended audience
This course is intended for C# developers with practical experience in the .NET framework.
▼Expand All
-
Module 1: C# Language Features
-
Developer Productivity
-
Implicit typing
-
Object and collection initializers
-
Automatic properties
-
Anonymous types
-
-
Extensibility Concepts
-
Extension methods
-
Partial methods
-
-
Functional Programming Features
-
Lambda expressions
-
Expression trees
-
-
LINQ Support Features
-
An overview of language query operators.
-
-
Lab 1: Extension Methods and IEnumerable<T>
-
ForEach extension method for IEnumerable<T>.
-
MaxElement extension method for IEnumerable<T>.
-
-
Lab 2: Extension Methods and Anonymous Types
-
Extension method for turning anonymous types into tuples.
-
-
-
Module 2: LINQ Query Operators
-
Motivation for Query Operators
-
Declarative vs. Imperative programming.
-
-
Developing Query Operators
-
C# 2.0 iterators
-
Deferred execution
-
-
Categorizing query operators
-
Filtering
-
Aggregation
-
Ordering
-
Grouping
-
Projection
-
miscellaneous operators
-
-
Language integration of query operators
-
Lab 3: Implementing Query Operators
-
Implementing LastOrDefault, Cast, Concat and Aggregate.
-
-
-
Module 3: Applied LINQ to Objects
-
Using Query Operators with Objects.
-
Customizing query operators
-
The query pattern
-
Examples of LINQ to Objects
-
LINQ to Reflection
-
LINQ to File System
-
LINQ to Strings
-
LINQ to WCF Contracts
-
-
Lab 4: Implementing the Query Pattern
-
Implementing the query pattern for the DataTable class.
-
-
Lab 5: Using LINQ Queries
-
Using LINQ to query the file system.
-
Using LINQ to query the .NET Reflection API.
-
-
-
Module 4: LINQ to XML
-
Introducing the XElement API
-
Constructing XML fragments with XElement
-
Querying XElement DOMs
-
Axis methods
-
Lab 6: LINQ to XML
-
XML query and transform using LINQ.
-
XML query and aggregation using LINQ to XML and LINQ to Objects.
-
-
-
Module 5: LINQ to SQL
-
LINQ to DataSet
-
Why DataSets are not enough.
-
-
LINQ to SQL as an Object-Relational Mapper
-
Mapping using attributes
-
mapping using external XML mapping files
-
mapping inheritance relationships
-
-
The Data Context
-
Queries
-
updates
-
inserts
-
deletes
-
stored procedures and database functions
-
custom SQL strings
-
database creation from schema
-
transactions
-
preloading and tracking data
-
optimistic locking and concurrency control
-
-
Visual Studio LINQ to SQL Designer vs. SQLMetal.
-
Lab 7: Integrating XML Mapping
-
Constructing a DataContext from embedded XML mapping files.
-
-
Lab 8: Testing Concurrency Control and Object Tracking
-
Transactional work and concurrency control.
-
Non-transactional work and conflict management.
-
Object tracking and identity management.
-
-
Lab 9: Inheritance Mapping
-
Mapping a hierarchy using attributes.
-
Creating a database from schema.
-
-
-
Module 6: Beyond LINQ
-
Overview of Parallel LINQ.
-
Overview of ADO.NET Entity Framework.
-
Overview of ADO.NET Data Services.
-
Overview of Custom Query Providers.
-
Performance Considerations of LINQ to Objects.
-
Performance Considerations of LINQ to XML.
-
Performance Considerations of LINQ to SQL.
-
- Working knowledge of C# 2.0: Generics, anonymous methods, iterators
- Working knowledge of the .NET framework: Collections, ADO.NET basics, XML
- Apply the new C# 3.0 language features in appropriate and practical scenarios
- Apply the LINQ framework for accessing various types of data sources
- Gain a general understanding of the LINQ extensibility model for writing query providers and customizing existing data sources
- Gain a general understanding of future frameworks in the LINQ area, including ADO.NET Data Services, ADO.NET Entity Framework, Parallel LINQ and other
Contact Us


SEND
Related Courses